Let us Read for you. 25 Linux Server Security and Hardening Tips: How can Secure Linux Server Everybody says that Linux is secure by default and agreed to some extent (It’s debatable topics). However, Linux has an in-built security model in place by default. Need to tune it up and customize as per your need […]
Vesta WEB API
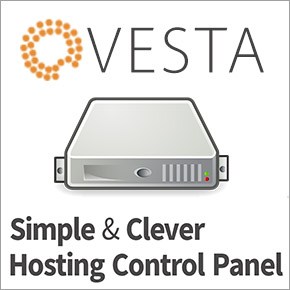
Let us Read for you.
|
Vesta WEB API is used to perform the functions of the control panel.You can use it internally to sync DNS clusters, to integrate WHMC billing system and to reset mail account passwords in Roundcube. Vesta API can be used for creating new user accounts, domains, databases. It can also build an alternative web interface.
This reference provides PHP code samples demonstrating how you can seamlessly integrate API into your application or script. However, you can use other languages to communicate with API.
- Create User Account
- Create Web/DNS/Mail Domain All Together
- Create Database
- List User Account
- List Web Domains
- Delete User Account
- Check Username and Password
- Return Codes
- Full Command List
Create User Account
<?php // Server credentials $vst_hostname = 'server.vestacp.com'; $vst_username = 'admin'; $vst_password = 'p4ssw0rd'; $vst_returncode = 'yes'; $vst_command = 'v-add-user'; // New Account $username = 'demo'; $password = 'd3m0p4ssw0rd'; $email = 'demo@gmail.com'; $package = 'default'; $fist_name = 'Rust'; $last_name = 'Cohle'; // Prepare POST query $postvars = array( 'user' => $vst_username, 'password' => $vst_password, 'returncode' => $vst_returncode, 'cmd' => $vst_command, 'arg1' => $username, 'arg2' => $password, 'arg3' => $email, 'arg4' => $package, 'arg5' => $fist_name, 'arg6' => $last_name ); $postdata = http_build_query($postvars); // Send POST query via cURL $postdata = http_build_query($postvars); $curl = curl_init(); curl_setopt($curl, CURLOPT_URL, 'https://' . $vst_hostname . ':8083/api/'); curl_setopt($curl, CURLOPT_RETURNTRANSFER,true); curl_setopt($curl, CURLOPT_SSL_VERIFYPEER, false); curl_setopt($curl, CURLOPT_SSL_VERIFYHOST, false); curl_setopt($curl, CURLOPT_POST, true); curl_setopt($curl, CURLOPT_POSTFIELDS, $postdata); $answer = curl_exec($curl); // Check result if($answer == 0) { echo "User account has been successfuly created\n"; } else { echo "Query returned error code: " .$answer. "\n"; } ?>
Add Web/DNS/Mail Domain
<?php // Server credentials $vst_hostname = 'server.vestacp.com'; $vst_username = 'admin'; $vst_password = 'p4ssw0rd'; $vst_returncode = 'yes'; $vst_command = 'v-add-domain'; // New Domain $username = 'demo'; $domain = 'demo.vestacp.com'; // Prepare POST query $postvars = array( 'user' => $vst_username, 'password' => $vst_password, 'returncode' => $vst_returncode, 'cmd' => $vst_command, 'arg1' => $username, 'arg2' => $domain ); $postdata = http_build_query($postvars); // Send POST query via cURL $postdata = http_build_query($postvars); $curl = curl_init(); curl_setopt($curl, CURLOPT_URL, 'https://' . $vst_hostname . ':8083/api/'); curl_setopt($curl, CURLOPT_RETURNTRANSFER,true); curl_setopt($curl, CURLOPT_SSL_VERIFYPEER, false); curl_setopt($curl, CURLOPT_SSL_VERIFYHOST, false); curl_setopt($curl, CURLOPT_POST, true); curl_setopt($curl, CURLOPT_POSTFIELDS, $postdata); $answer = curl_exec($curl); // Check result if($answer == 0) { echo "Domain has been successfuly created\n"; } else { echo "Query returned error code: " .$answer. "\n"; } ?>
Create Database
<?php // Server credentials $vst_hostname = 'server.vestacp.com'; $vst_username = 'admin'; $vst_password = 'p4ssw0rd'; $vst_returncode = 'yes'; $vst_command = 'v-add-database'; // New Database $username = 'demo'; $db_name = 'wordpress'; $db_user = 'wordpress'; $db_pass = 'wpbl0gp4s'; // Prepare POST query $postvars = array( 'user' => $vst_username, 'password' => $vst_password, 'returncode' => $vst_returncode, 'cmd' => $vst_command, 'arg1' => $username, 'arg2' => $db_name, 'arg3' => $db_user, 'arg4' => $db_pass ); $postdata = http_build_query($postvars); // Send POST query via cURL $curl = curl_init(); curl_setopt($curl, CURLOPT_URL, 'https://' . $vst_hostname . ':8083/api/'); curl_setopt($curl, CURLOPT_RETURNTRANSFER,true); curl_setopt($curl, CURLOPT_SSL_VERIFYPEER, false); curl_setopt($curl, CURLOPT_SSL_VERIFYHOST, false); curl_setopt($curl, CURLOPT_POST, true); curl_setopt($curl, CURLOPT_POSTFIELDS, $postdata); $answer = curl_exec($curl); // Check result if($answer == 0) { echo "Database has been successfuly created\n"; } else { echo "Query returned error code: " .$answer. "\n"; } ?>
List User Account
<?php // Server credentials $vst_hostname = 'server.vestacp.com'; $vst_username = 'admin'; $vst_password = 'p4ssw0rd'; $vst_command = 'v-list-user'; // Account $username = 'demo'; $format = 'json'; // Prepare POST query $postvars = array( 'user' => $vst_username, 'password' => $vst_password, 'cmd' => $vst_command, 'arg1' => $username, 'arg2' => $format ); $postdata = http_build_query($postvars); // Send POST query via cURL $postdata = http_build_query($postvars); $curl = curl_init(); curl_setopt($curl, CURLOPT_URL, 'https://' . $vst_hostname . ':8083/api/'); curl_setopt($curl, CURLOPT_RETURNTRANSFER,true); curl_setopt($curl, CURLOPT_SSL_VERIFYPEER, false); curl_setopt($curl, CURLOPT_SSL_VERIFYHOST, false); curl_setopt($curl, CURLOPT_POST, true); curl_setopt($curl, CURLOPT_POSTFIELDS, $postdata); $answer = curl_exec($curl); // Parse JSON output $data = json_decode($answer, true); // Print result print_r($data); ?>
List Web Domains
<?php // Server credentials $vst_hostname = 'server.vestacp.com'; $vst_username = 'admin'; $vst_password = 'p4ssw0rd'; $vst_command = 'v-list-web-domain'; // Account $username = 'demo'; $domain = 'demo.vestacp.com'; $format = 'json'; // Prepare POST query $postvars = array( 'user' => $vst_username, 'password' => $vst_password, 'cmd' => $vst_command, 'arg1' => $username, 'arg2' => $domain, 'arg3' => $format ); $postdata = http_build_query($postvars); // Send POST query via cURL $postdata = http_build_query($postvars); $curl = curl_init(); curl_setopt($curl, CURLOPT_URL, 'https://' . $vst_hostname . ':8083/api/'); curl_setopt($curl, CURLOPT_RETURNTRANSFER,true); curl_setopt($curl, CURLOPT_SSL_VERIFYPEER, false); curl_setopt($curl, CURLOPT_SSL_VERIFYHOST, false); curl_setopt($curl, CURLOPT_POST, true); curl_setopt($curl, CURLOPT_POSTFIELDS, $postdata); $answer = curl_exec($curl); // Parse JSON output $data = json_decode($answer, true); // Print result print_r($data); ?>
Delete User Account
<?php // Server credentials $vst_hostname = 'server.vestacp.com'; $vst_username = 'admin'; $vst_password = 'p4ssw0rd'; $vst_returncode = 'yes'; $vst_command = 'v-delete-user'; // Account $username = 'demo'; // Prepare POST query $postvars = array( 'user' => $vst_username, 'password' => $vst_password, 'returncode' => $vst_returncode, 'cmd' => $vst_command, 'arg1' => $username ); $postdata = http_build_query($postvars); // Send POST query via cURL $postdata = http_build_query($postvars); $curl = curl_init(); curl_setopt($curl, CURLOPT_URL, 'https://' . $vst_hostname . ':8083/api/'); curl_setopt($curl, CURLOPT_RETURNTRANSFER,true); curl_setopt($curl, CURLOPT_SSL_VERIFYPEER, false); curl_setopt($curl, CURLOPT_SSL_VERIFYHOST, false); curl_setopt($curl, CURLOPT_POST, true); curl_setopt($curl, CURLOPT_POSTFIELDS, $postdata); $answer = curl_exec($curl); // Check result if($answer == 0) { echo "User account has been successfuly deleted\n"; } else { echo "Query returned error code: " .$answer. "\n"; } ?>
Check Username and Password
<?php // Server credentials $vst_hostname = 'server.vestacp.com'; $vst_username = 'admin'; $vst_password = 'p4ssw0rd'; $vst_command = 'v-check-user-password'; $vst_returncode = 'yes'; // Account $username = 'demo'; $password = 'demopassword'; // Prepare POST query $postvars = array( 'user' => $vst_username, 'password' => $vst_password, 'cmd' => $vst_command, 'arg1' => $username, 'arg2' => $password ); $postdata = http_build_query($postvars); // Send POST query via cURL $postdata = http_build_query($postvars); $curl = curl_init(); curl_setopt($curl, CURLOPT_URL, 'https://' . $vst_hostname . ':8083/api/'); curl_setopt($curl, CURLOPT_RETURNTRANSFER,true); curl_setopt($curl, CURLOPT_SSL_VERIFYPEER, false); curl_setopt($curl, CURLOPT_SSL_VERIFYHOST, false); curl_setopt($curl, CURLOPT_POST, true); curl_setopt($curl, CURLOPT_POSTFIELDS, $postdata); $answer = curl_exec($curl); // Check result if($answer == 0) { echo "OK: User can login\n"; } else { echo "Error: Username or password is incorrect\n"; } ?>
Comments (0)